EDB simple example#
This example shows how to use the EDBCommon
class to open an existing EDB project.
Perform required imports#
Perform the required imports.
[1]:
import os
import shutil
[2]:
from ansys.aedt.core import generate_unique_folder_name
[3]:
from ansys.aedt.toolkits.common.utils import download_file
from ansys.aedt.toolkits.common.backend.api import EDBCommon
Initialize temporary folder and project settings#
Initialize a temporary folder to copy the input file into and specify project settings.
[4]:
URL_BASE = "https://raw.githubusercontent.com/ansys/example-data/master/toolkits/common/"
EDB_PROJECT = "edb_edge_ports.aedb/edb.def"
URL = os.path.join(URL_BASE, EDB_PROJECT)
temp_folder = os.path.join(generate_unique_folder_name())
edb_path = os.path.join(temp_folder, "edb_example.aedb")
os.makedirs(edb_path, exist_ok=True)
local_project = os.path.join(edb_path, "edb.def")
download_file(URL, local_project)
[4]:
'C:\\Users\\ansys\\AppData\\Local\\Temp\\pyaedt_prj_55W\\edb_example.aedb\\edb.def'
Initialize toolkit#
Initialize the toolkit.
[5]:
toolkit = EDBCommon()
Initialize EDB project#
Open the EDB project.
[6]:
load_edb_msg = toolkit.load_edb(edb_path)
Get toolkit properties#
Get toolkit properties, which contain the project information.
[7]:
new_properties = toolkit.get_properties()
edb_project = new_properties["active_project"]
Save project#
Copy the current project in a new file.
[8]:
directory, old_file_name = os.path.split(edb_project)
new_path = os.path.join(directory, "new_edb.aedb")
toolkit.save_edb(new_path)
INFO - Project C:\Users\ansys\AppData\Local\Temp\pyaedt_prj_55W\new_edb.aedb saved
[8]:
True
Get cell names#
Get cell names using PyEDB.
[9]:
toolkit.logger.info("Play with EDB")
cell_names = toolkit.edb.cell_names
toolkit.edb.nets.plot()
INFO - Play with EDB
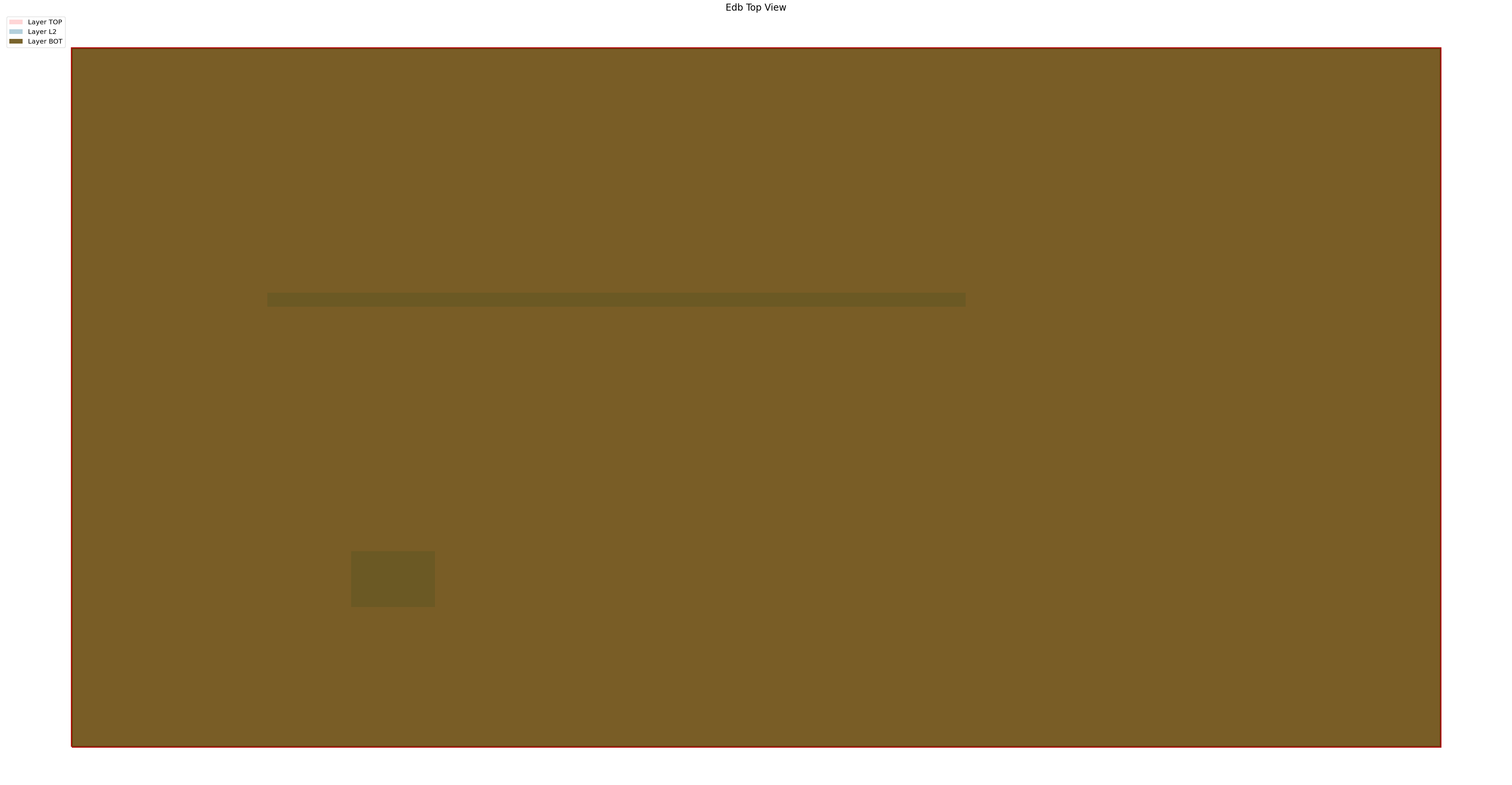
[9]:
(<Figure size 6000x3000 with 1 Axes>,
<Axes: title={'center': 'Edb Top View EMDesign1'}>)
Save and release EDB#
Save and release EDB.
[10]:
toolkit.close_edb()
INFO - EDB is closed.
[10]:
True
Remove temporary folder#
Remove the temporary folder.
[11]:
shutil.rmtree(temp_folder, ignore_errors=True)